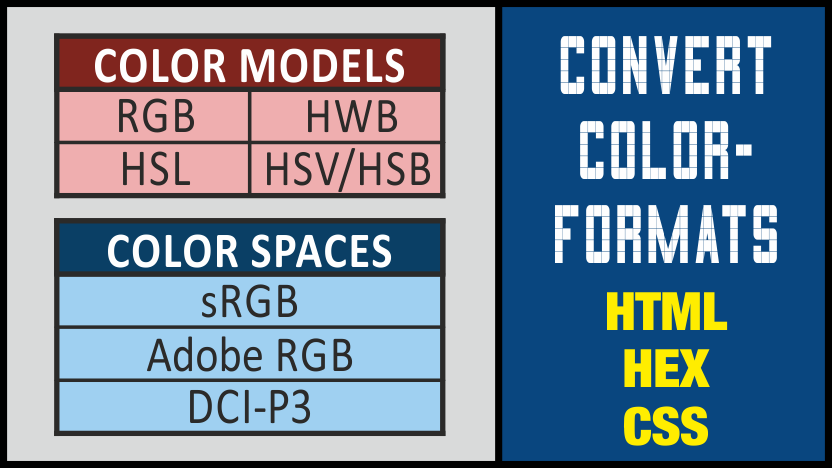
This article describes the difference between color spaces and color models and explains how different color formats can be converted into each other.
Table of contents
The difference between color models and color spaces
The two terms color model and color space are often confused with each other, but in fact they must be distinguished from each other. In principle, a color model represents the basic framework, i.e. the coordinate system in mathematical terms. The best known of these is probably the RGB color model: with the help of three dimensions, the individual color is described from the three primary colors red (R), green (G) and blue (B). The whole thing can be visualized as a cube, where the height, width and depth reflect the respective color component. Most color models consist of three or four dimensions.
For a color model, i.e. the specific coordinate system, there are different color spaces that refer to this color model. This simply means that each numerical value of the color model is assigned a specific color. The best-known color space and de facto standard on the Internet is the sRGB color space, which is based on the RGB color model.
Different color models
The most common color models RGB, HSL, HSV/HSB, HWB and YCMK are presented below.
RGB color model
As already mentioned in the introduction, the RGB color model consists of the three basic colors red (R), green (G) and blue (B). A specific color is always a mixture of these three components. This is an additive color system in which the colors are generated using emitted light. The intensity of each of these colors is usually described with a number from 0 to 255. Without a specific color space, however, this RGB value initially has no meaning, as only the selection of the color space assigns specific colors to the individual values. Graphically, this can be imagined as a cube in which each dimension corresponds to a different RGB channel. Mathematically, we are talking here about a Cartesian coordinate system.

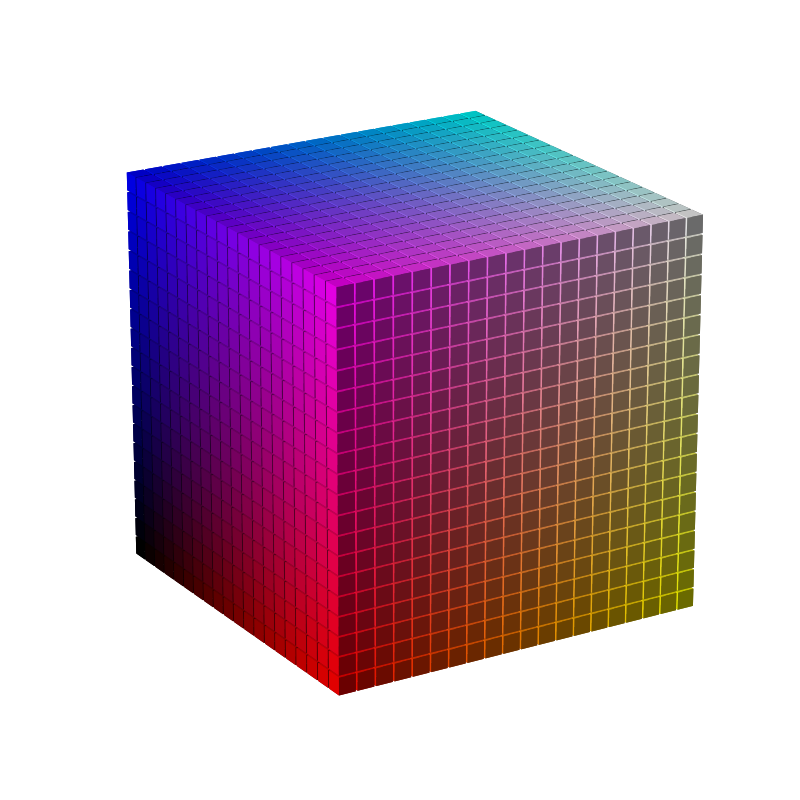
HSL color model
This color model uses the three components Hue (H:), Saturation (S) and Lightness (L). You can imagine this either correctly as a cylinder or somewhat more intuitively as a double color cone. The Hue (H) is described as an angle on the color circle from 0° to 360°. The Saturation (S), on the other hand, is usually specified as a percentage from 0 to 100 and decreases from the edge of the color wheel to the middle, i.e. the Hue is strong at the edge and becomes weaker towards the middle, ending in a gray value in the middle. The color brightness is also normally given as a percentage. The value 0 corresponds to the lower tip of the double cone (black) and the value 100 represents the upper tip (white). It can therefore be seen that a color brightness of 50% must be set for maximum saturation in the HSL color model. The color cone as a concept is more intuitive because all colors converge towards black with increasing darkness and towards white with increasing brightness.
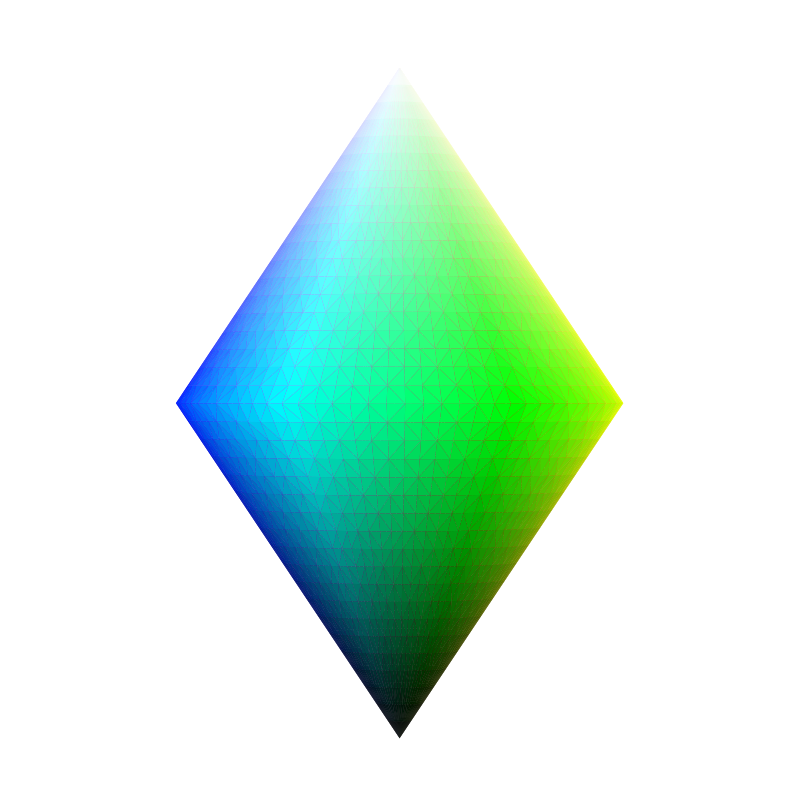
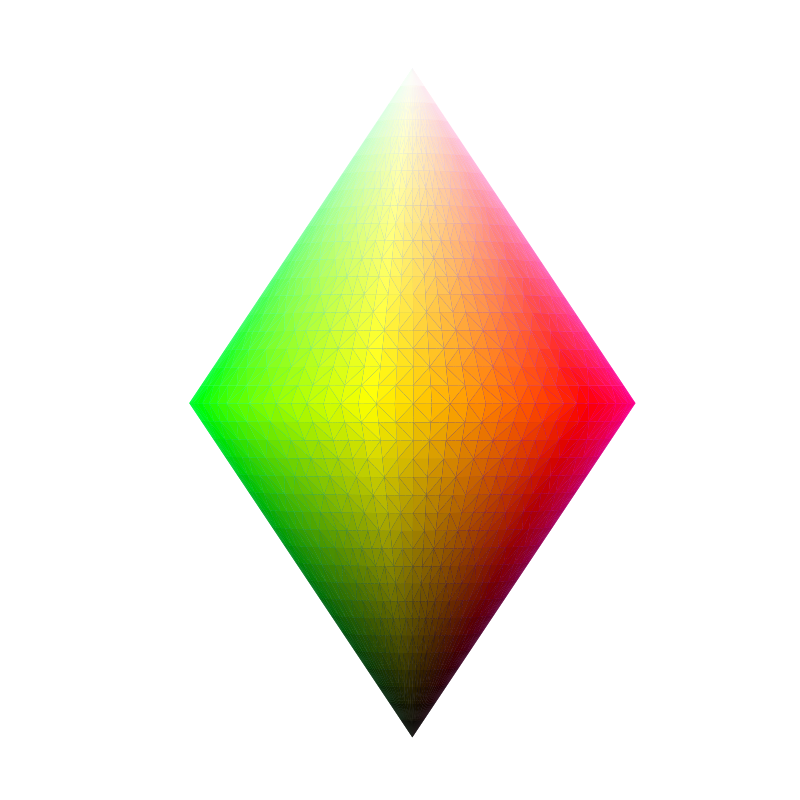
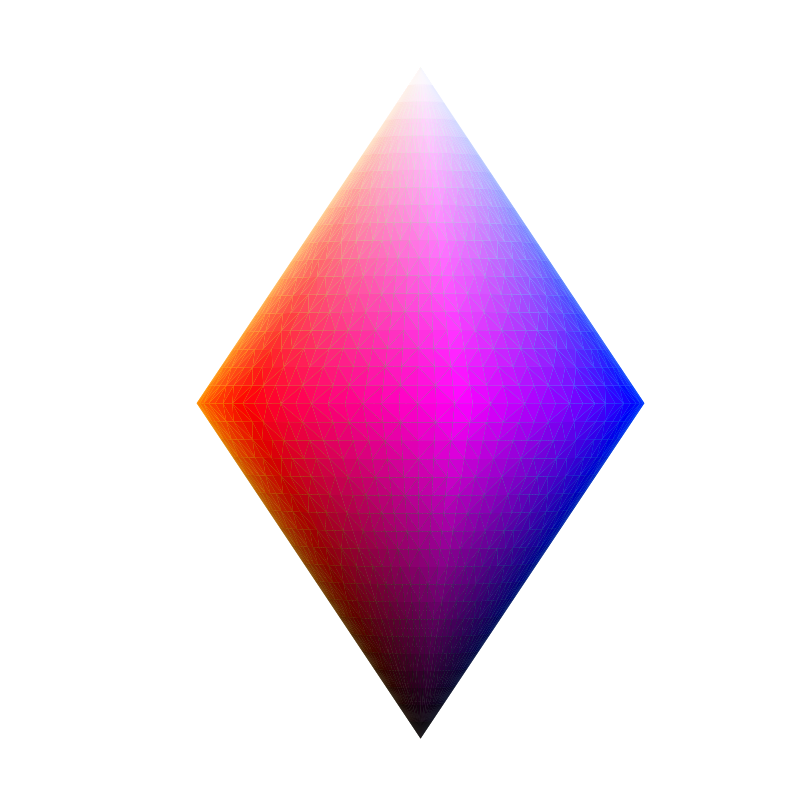
HSV/HSB color model
The HSV or HSB color model is similar to the HSL color model. The H also stands for the Hue, the S for the Saturation and the V or B for the Brightness. In contrast to the HSL model, we have to imagine the HSV/HSB color model as a simple upside-down cone. The maximum saturation of the colors is not in the middle at 50 %, but at the very top at 100 %. At the top in the middle is the color white and towards the bottom all colors converge towards black.
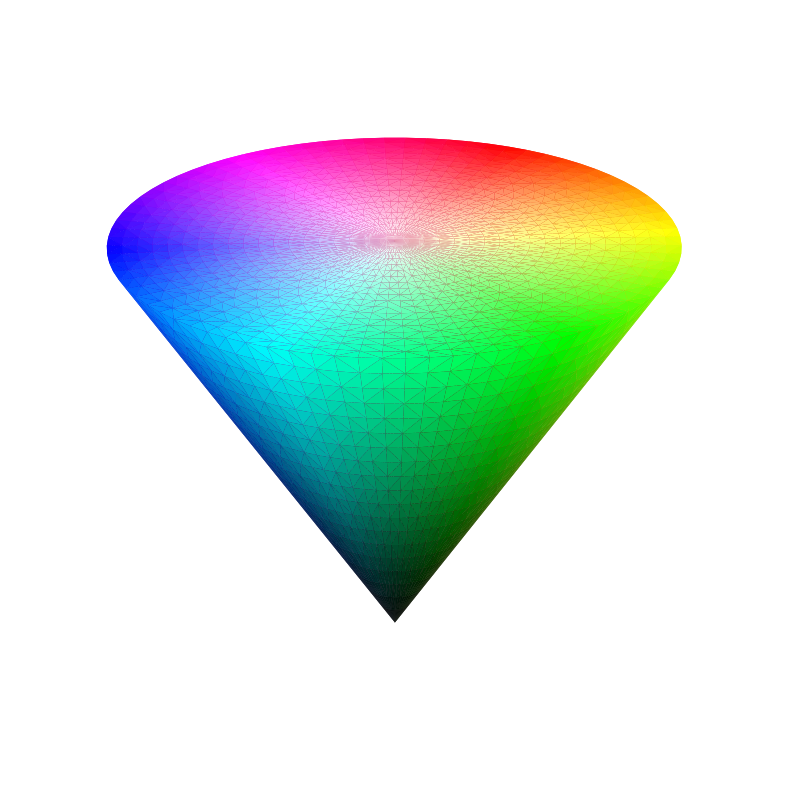
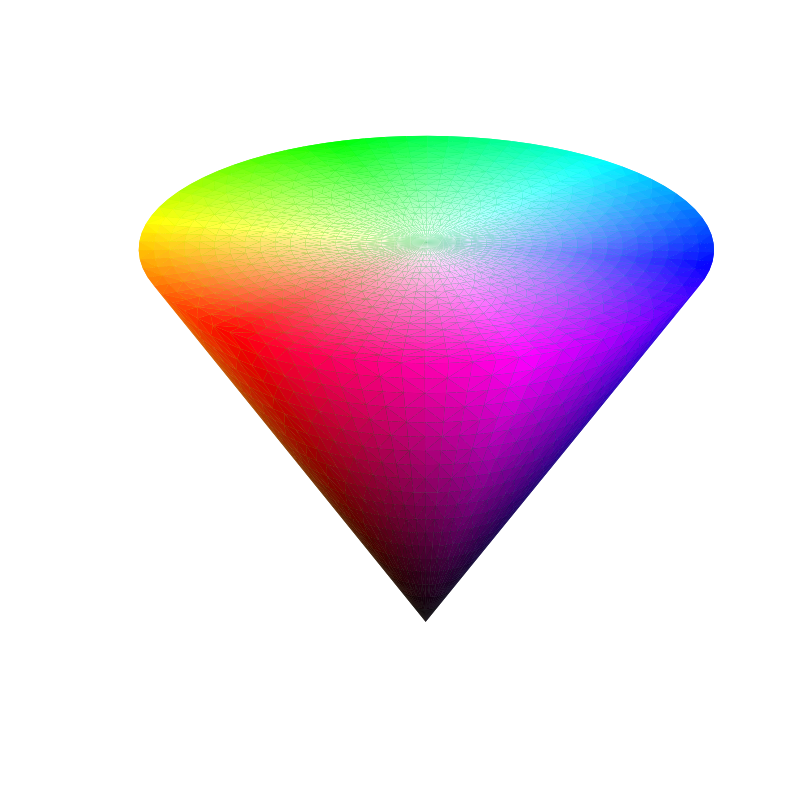
HWB color model
The HWB color model is also similar to the two models described above. H still stands for the Hue and the other two components are the Whiteness (W) and the Blackness (B). If we imagine this as a cone shape again, then the edge of the base is white, the colors are strongest in the middle of the base and the colors change to black towards the top. In fact, the cone shape is more accurate here than a cylinder, because the sum of the white and black components may not exceed 100% or will be scaled down accordingly when converting to another color model if the value is exceeded.
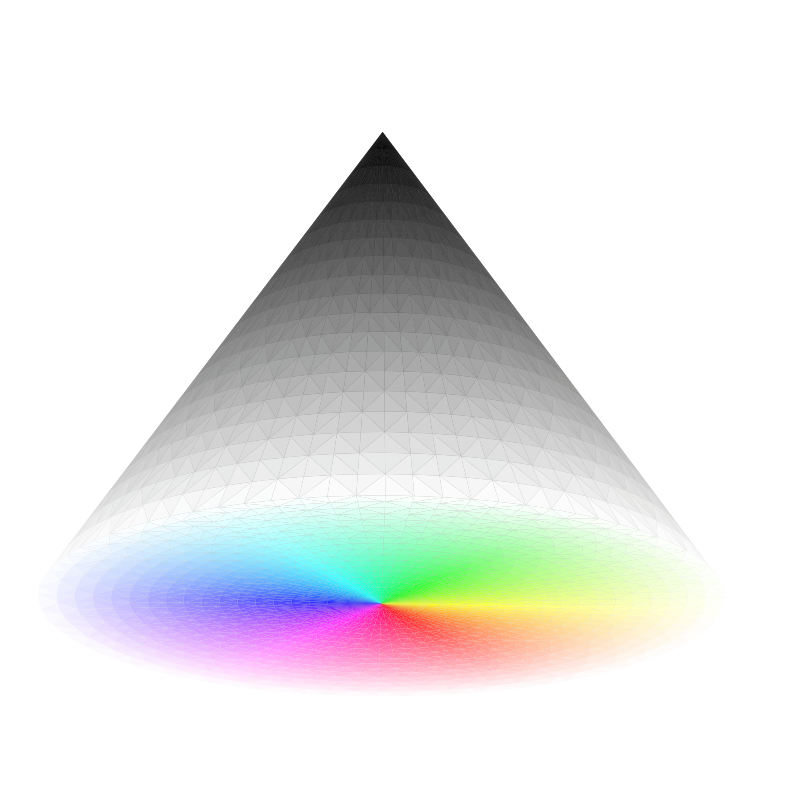
CMYK color model
The CMYK color model is a subtractive color system that is used for printing. Subtractive means that the colors are applied to a surface (e.g. paper) and the more of them are applied, the more reflective light is “swallowed”, i.e. the darker it becomes. CMYK stands for the components Cyan (C), Magenta (M), Yellow (Y) and Black (K: Key). The value range of the individual components is from 0 to 100 %. If 0 % is applied to a white paper, then - oh wonder - it is white. With 100 % of the three basic CMY colors, a black is created in principle, but only in principle. In reality, this is not as deep a black as you would like, so there is the additional black (K), which is usually cheaper than mixing it from the three basic colors.
In contrast to the other color models presented so far, it is not possible to convert a color from or to the CMYK system without further information. Some websites do offer this, but the result is usually not at all useful, as the conversion depends on the respective printing process. For a meaningful conversion, you need standardized data sets, the so-called ICC color profiles.
Different color spaces
A color space assigns specific colors to the numerical values of a color model. In addition to the sRGB color space, the de facto standard on the Internet, there are numerous other color spaces. The best known are probably the Adobe RGB color space and the DCI-P3 color space.
sRGB color space
The sRGB color space is mainly used for displaying images on screens. This color space was developed in the 1990s to enable uniform color reproduction on different devices. It is important to understand that the sRGB color space can only display around a third of the colors that our eyes can distinguish, i.e. there are colors that are not visible on the monitor.
Adobe-RGB color space
The Adobe RGB color space was developed for professional image applications, such as prepress or photography. Both sRGB and Adobe RGB can display the same number of colors, but the Adobe RGB color space can display a wider spectrum, especially in the blue and green range. If your computer monitor or mobile device is capable of displaying this color space, then it can basically show you colors that sRGB cannot. However, if your display device is only capable of sRGB anyway, then it is impossible to actually show you the difference.
DCI-P3 color space
Another color space is the DCI-P3 color space, which covers a comparably large area to the Adobe RGB color space, although it is somewhat red-shifted in comparison. This color space was originally developed for the film and cinema industry.
Conversion of color models in PHP
The color format converter implemented on this page performs various internal conversions in the PHP programming language. The specific conversion algorithms are presented below for all programming enthusiasts.
Convert RGB to HEX
//---------------------------------------------------------------------------------------------------------------- /*! \brief Convert RGB to HEX \param[in] $o_R Red value [0,255] \param[in] $o_G Green value [0,255] \param[in] $o_B Blue value [0,255] \return String with HEX value (e.g. #123456) */ //---------------------------------------------------------------------------------------------------------------- function rgb_to_hex($o_R, $o_G, $o_B) { $R = sprintf("%02x", $o_R); $G = sprintf("%02x", $o_G); $B = sprintf("%02x", $o_B); return strtoupper("#".$R.$G.$B); }
Convert RGB to HSV
//---------------------------------------------------------------------------------------------------------------- /*! \brief Convert RGB to HSV \param[in] $o_R Red value [0,255] \param[in] $o_G Green value [0,255] \param[in] $o_B Blue value [0,255] \return Array with HSV values (H: [0,360], S: [0,100], V: [0,100]) */ //---------------------------------------------------------------------------------------------------------------- function rgb_to_hsv($o_R, $o_G, $o_B) { $r = $o_R / 255; $g = $o_G / 255; $b = $o_B / 255; $min_RGB = min($r, $g, $b); $max_RGB = max($r, $g, $b); $delta = $max_RGB - $min_RGB; if ($delta == 0) // R = G = B { $H = 0; $S = 0; } else { if ($r == $max_RGB) { $H = 60 * (($g - $b) / $delta); if ($H < 0) { $H += 360; } } else if ($g == $max_RGB) { $H = 60 * (2 + (($b - $r) / $delta)); } else { $H = 60 * (4 + (($r - $g) / $delta)); } $S = 100 * ($delta / $max_RGB); } // V is simply the maximum value of R, G, B $V = 100 * $max_RGB; return array( "H" => $H, "S" => $S, "V" => $V ); }
Convert RGB to HSL
//---------------------------------------------------------------------------------------------------------------- /*! \brief Convert RGB to HSL \param[in] $o_R Red value [0,255] \param[in] $o_G Green value [0,255] \param[in] $o_B Blue value [0,255] \return Array with HSL values (H: [0,360], S: [0,100], L: [0,100]) */ //---------------------------------------------------------------------------------------------------------------- function rgb_to_hsl($o_R, $o_G, $o_B) { $r = $o_R / 255; $g = $o_G / 255; $b = $o_B / 255; $min_RGB = min($r, $g, $b); $max_RGB = max($r, $g, $b); $delta = $max_RGB - $min_RGB; if ($delta == 0) // R = G = B { $H = 0; $S = 0; } else { if ($r == $max_RGB) { $H = 60 * (($g - $b) / $delta); if ($H < 0) { $H += 360; } } else if ($g == $max_RGB) { $H = 60 * (2 + (($b - $r) / $delta)); } else { $H = 60 * (4 + (($r - $g) / $delta)); } $S = 100 * ($delta / (1 - abs($min_RGB + $max_RGB - 1))); } $L = 100 * ($min_RGB + $max_RGB) / 2; return array( "H" => $H, "S" => $S, "L" => $L, ); }
Convert HSV to RGB
//---------------------------------------------------------------------------------------------------------------- /*! \brief Convert HSV to RGB \param[in] $o_H Hue [0,360] \param[in] $o_S Saturation [0,100] \param[in] $o_V Value [0,100] \return Array with RGB values (R: [0,255], G: [0,255], B: [0,255]) */ //---------------------------------------------------------------------------------------------------------------- function hsv_to_rgb($o_H, $o_S, $o_V) { $H = $o_H; $s = $o_S / 100; $v = $o_V / 100; $hi = floor($H / 60); $f = ($H / 60) - $hi; $p = $v * (1 - $s); $q = $v * (1 - ($s * $f)); $t = $v * (1 - ($s * (1 - $f))); switch ($hi) { case 1: $r = $q; $g = $v; $b = $p; break; case 2: $r = $p; $g = $v; $b = $t; break; case 3: $r = $p; $g = $q; $b = $v; break; case 4: $r = $t; $g = $p; $b = $v; break; case 5: $r = $v; $g = $p; $b = $q; break; default: $r = $v; $g = $t; $b = $p; break; } $R = 255 * $r; $G = 255 * $g; $B = 255 * $b; return array( "R" => $R, "G" => $G, "B" => $B, ); }
Convert HSV to HSL
//---------------------------------------------------------------------------------------------------------------- /*! \brief Convert HSV to HS \param[in] $o_H Hue [0,360] \param[in] $o_S Saturation [0,100] \param[in] $o_V Value [0,100] \return Array with HSL values (H: [0,360], S: [0,100], L: [0,100]) */ //---------------------------------------------------------------------------------------------------------------- function hsv_to_hsl($o_H, $o_S, $o_V) { $HSV_H = $o_H; $HSV_s = $o_S / 100; $HSV_v = $o_V / 100; $HSL_H = $HSV_H; $HSL_l = $HSV_v * (1 - ($HSV_s / 2)); if (($HSL_l == 0) || ($HSL_l == 1)) { $HSL_s = 0; } else { $HSL_s = ($HSV_v - $HSL_l) / min($HSL_l, (1 - $HSL_l)); } $HSL_S = 100 * $HSL_s; $HSL_L = 100 * $HSL_l; return array( "H" => $HSL_H, "S" => $HSL_S, "L" => $HSL_L, ); }
Convert HSL to HSV
//---------------------------------------------------------------------------------------------------------------- /*! \brief Convert HSL to HSV \param[in] $o_H Hue [0,360] \param[in] $o_S Saturation [0,100] \param[in] $o_L Lightness [0,100] \return Array with HSV values (H: [0,360], S: [0,100], V: [0,100]) */ //---------------------------------------------------------------------------------------------------------------- function hsl_to_hsv($o_H, $o_S, $o_L) { $HSL_H = $o_H; $HSL_s = $o_S / 100; $HSL_l = $o_L / 100; $HSV_H = $HSL_H; $HSV_v = $HSL_l + ($HSL_s * min($HSL_l, (1 - $HSL_l))); if ($HSV_v == 0) { $HSV_s = 0; } else { $HSV_s = 2 * (1 - ($HSL_l / $HSV_v)); } $HSV_S = 100 * $HSV_s; $HSV_V = 100 * $HSV_v; return array( "H" => $HSV_H, "S" => $HSV_S, "V" => $HSV_V, ); }
Convert HSV to HWB
//---------------------------------------------------------------------------------------------------------------- /*! \brief Convert HSV to HWB \param[in] $o_H Hue [0,360] \param[in] $o_S Saturation [0,100] \param[in] $o_V Value [0,100] \return Array with HWB values (H: [0,360], W: [0,100], B: [0,100]) */ //---------------------------------------------------------------------------------------------------------------- function hsv_to_hwb($o_H, $o_S, $o_V) { $HSV_H = $o_H; $HSV_s = $o_S / 100; $HSV_v = $o_V / 100; $HWB_H = $HSV_H; $HWB_w = (1 - $HSV_s) * $HSV_v; $HWB_b = 1 - $HSV_v; $HWB_W = 100 * $HWB_w; $HWB_B = 100 * $HWB_b; return array( "H" => $HWB_H, "W" => $HWB_W, "B" => $HWB_B, ); }
Convert HWB to HSV
//---------------------------------------------------------------------------------------------------------------- /*! \brief Convert HWB to HSV Precondition: Whiteness + Blackness < 100% \param[in] $o_H Hue [0,360] \param[in] $o_W Whiteness [0,100] \param[in] $o_B Blackness [0,100] \return Array with HSV values (H: [0,360], S: [0,100], V: [0,100]) */ //---------------------------------------------------------------------------------------------------------------- function hwb_to_hsv($o_H, $o_W, $o_B) { $HWB_H = $o_H; $HWB_w = $o_W / 100; $HWB_b = $o_B / 100; $HSV_H = $HWB_H; $HSV_v = 1 - $HWB_b; if ($HWB_b < 1) { $HSV_s = 1 - ($HWB_w / (1 - $HWB_b)); } else { $HSV_s = 0; } $HSV_S = 100 * $HSV_s; $HSV_V = 100 * $HSV_v; return array( "H" => $HSV_H, "S" => $HSV_S, "V" => $HSV_V, ); }
Conclusion
We now know the difference between color models and color spaces. The most important examples of these were presented and for the technically interested among you, the specific functions used for conversion in the color converter provided on this page were shown.
TO THE COLOR FORMAT CONVERTER